By Tim McVinish
‘Tis the season for festive websites
Well, it’s the holiday season. While our homes, shopping centres, and everything else around us gets a sprinkle of holiday magic, websites are often overlooked. So today we’re going to explore creating a simple script that can be used to add a little festive flare to any website. This script will display a wreath and countdown to Christmas that, when clicked, triggers a whimsical snowflake animation. Let’s dive into the details!
Getting your digital decorating kit ready
Before we start decking the digital halls, let’s run through everything needed to add some festive flair to your website. You’ll need:
Essential tools
- Access to your website’s code or the ability to add a script (more on that later)
- A code editor
- Basic understanding of HTML, CSS, and JavaScript
- Holiday-themed images (PNG files with transparent backgrounds are preferred). We’re using a wreath.
- Somewhere to host the image so our script can reference it
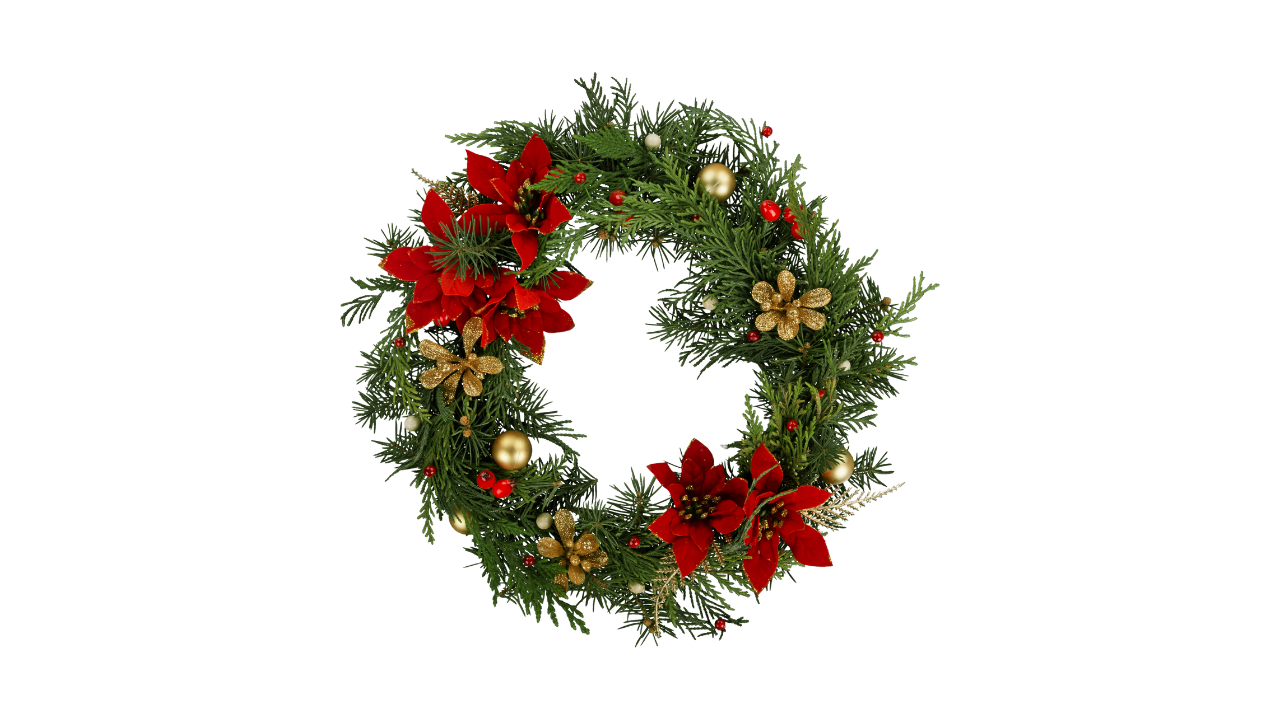
Step 1: Pulling out the digital decos
Just like with decorating the house, lets first open our cupboard and dig out our decorations. Personally I’m a minimalist, so we’ll keep things simple for this demo. Feel free to go as over-the-top-tacky-tinsel-too-many-fairy-lights-festive as you desire though.
const wrapper = document.createElement('div');
wrapper.id = 'the-wrapping';
const image = document.createElement('img');
image.id = 'digital-decoration';
image.src = '../../../assets/img/xmas-decoration-trimmed.png';
const countdown = document.createElement('div');
countdown.id = 'christmas-countdown';
countdown.textContent = 25 - new Date().getDate();
wrapper.appendChild(image);
wrapper.appendChild(countdown);
document.body.appendChild(wrapper);
Here we’ve got a wrapper to contain everything, a png of a Christmas wreath, and a simple countdown display the sleeps till Christmas.
Step 2: Hanging the garlands
We’ll use a dash of super special Christams CSS to place our decorations on the page. This will include styles, positioning and animations. Again, feel free to add your own spin and create your own winter wonderland.
First, we need to define the styles for our Christmas-themed elements. This includes positioning, animations, and responsive design. Here’s how you can do it:
const styles = document.createElement('style');
styles.textContent = `
#the-wrapping {
position: fixed;
display: flex;
align-items: center;
justify-content: center;
bottom: 50px; // position as you desire
right: 50px; // position as you desire
width: 120px; // size as you desire
height: 120px; // size as you desire
text-align: center;
font-size: 1rem;
background-color: rgba(255, 255, 255, 0.9);
box-shadow: 0 0 15px rgba(0, 0, 0, 0.1);
border-radius: 50%;
z-index: 1001;
cursor: pointer;
user-select: none;
}
#digital-decoration {
position: absolute;
top: 2px;
left: 6px;
width: 114px;
z-index: 1001;
animation: sway 2s ease-in-out infinite;
}
#christmas-countdown {
width: 118px;
font-size: 2rem;
font-weight: bold;
color: #333;
}
@keyframes sway {
0%, 100% { transform: rotate(-5deg); }
50% { transform: rotate(5deg); }
}
.snowflake {
position: fixed;
pointer-events: none;
animation: fall linear forwards;
z-index: 1000;
}
@keyframes fall {
to {
transform: translateY(100vh);
opacity: 0;
}
}
`;
document.head.appendChild(styles);
Step 3: A sprinkle of javascript magic
Those with a keen eye may have noticed the snowflake class in our css. “But there were no snowflakes in step 1!?”. This is for a final touch, a fun little click animation that creates a snow shower upon clicking our festive finishings. With that said, let’s add a listener for click events on our decorations and then trigger a createSnowflake function.
const christmasDecoration = document.getElementById('the-wrapping');
christmasDecoration.addEventListener('click', () => {
// Create snowflake effect
for (let i = 0; i < 10; i++) {
createSnowflake();
}
});
function createSnowflake() {
const snowflake = document.createElement('div');
snowflake.innerHTML = '❄';
snowflake.className = 'snowflake';
snowflake.style.left = `${christmasDecoration.offsetLeft + Math.random() * 110}px`;
snowflake.style.top = `${christmasDecoration.offsetTop}px`;
snowflake.style.fontSize = `${Math.random() * 10 + 10}px`;
snowflake.style.animationDuration = `${Math.random() * 8 + 1}s`;
document.body.appendChild(snowflake);
// Remove snowflake after animation
setTimeout(() => {
snowflake.remove();
}, 3000);
}
Now, when our decorations are clicked a flurry of frosty flakes will float over our site. Magical!
Step 4: Spread the Christmas cheer
Now, how do we deploy this? Well that will depend on where your site lives. If you have access to your code base then you can simply wrap the script in some <script>
tags and paste it where you please. If you’re using a platform like WordPress, Wix, or Squarespace, then you should be able to inject a script into your site via your dashboard. Below are some guides for these platforms. If you’re using a different platform Google should be able to help you out. Most platforms will provide a way to inject scripts.
And that’s a wrap! Please feel free to share any magical creations or alterations you come up with.
Guides for adding scripts to popular website platforms:
The complete script
(function () {
// content
const wrapper = document.createElement('div');
wrapper.id = 'the-wrapping';
const image = document.createElement('img');
image.id = 'digital-decoration';
image.src = './path-to-your-image/your-image.png'; // replace with the path
to your image
const countdown = document.createElement('div');
countdown.id = 'christmas-countdown';
countdown.textContent = 25 - new Date().getDate();
wrapper.appendChild(image);
wrapper.appendChild(countdown);
document.body.appendChild(wrapper);
// styles
const styles = document.createElement('style');
styles.textContent = `
#the-wrapping {
position: fixed;
display: flex;
align-items: center;
justify-content: center;
bottom: 50px;
right: 50px;
width: 120px;
height: 120px;
text-align: center;
font-size: 1rem;
background-color: rgba(255, 255, 255, 0.9);
box-shadow: 0 0 15px rgba(0, 0, 0, 0.1);
border-radius: 50%;
z-index: 1001;
cursor: pointer;
user-select: none;
}
#digital-decoration {
position: absolute;
top: 2px;
left: 6px;
width: 114px;
z-index: 1001;
animation: sway 2s ease-in-out infinite;
}
#christmas-countdown {
width: 118px;
font-size: 2rem;
font-weight: bold;
color: #333;
}
@keyframes sway {
0%, 100% { transform: rotate(-5deg); }
50% { transform: rotate(5deg); }
}
.snowflake {
position: fixed;
pointer-events: none;
animation: fall linear forwards;
z-index: 1000;
}
@keyframes fall {
to {
transform: translateY(100vh);
opacity: 0;
}
}
`;
document.head.appendChild(styles);
// functionality
const christmasDecoration = document.getElementById('the-wrapping');
christmasDecoration.addEventListener('click', () => {
// Create snowflake effect
for (let i = 0; i < 10; i++) {
createSnowflake();
}
});
function createSnowflake() {
const snowflake = document.createElement('div');
snowflake.innerHTML = '❄';
snowflake.className = 'snowflake';
snowflake.style.left = `${christmasDecoration.offsetLeft + Math.random()
* 110}px`;
snowflake.style.top = `${christmasDecoration.offsetTop}px`;
snowflake.style.fontSize = `${Math.random() * 10 + 10}px`;
snowflake.style.animationDuration = `${Math.random() * 8 + 1}s`;
document.body.appendChild(snowflake);
// Remove snowflake after animation
setTimeout(() => {
snowflake.remove();
}, 3000);
}
})();